If you don’t want to specific information in the URL being passed from tracking pixels to particular, there is a strategy that may work for your situation.
Why?
Sometimes there is sensitive information that platforms may flag, such as Facebook and Sensitive Health Information that a site may be sending along to Facebook. A common example is when a user types a search query and it contains a specific health ailment. The website (depending on the implementation) can capture this information in the query string to perform the search.

Like: https://mysite.com/search/?s=sensitiveinformationquery
Where the parameter `s` is set to sensitiveinformationquery which represents some private information you don’t want to track.
Otherwise, there may be just some information in the URL you’d rather not pass along to specific platforms in the URL.
General Idea
This example revolves around Facebook Pixel and Google Analytics calls within GTM.
GTM in this example is useful because based on the sequence of events, we can prioritize when specific pieces of code fire. We want the redacting code to fire before all tracking pixels so it can clean the information before pixels can pick it up the URL.
The general idea is:
- The user visits a URL with some sensitive information in the ‘s’ parameter (e.g. https://mysite.com/search/?s=sensitiveinformationquery)
- The redacting JS code in GTM runs early in the process and strips the s parameter from the url (replaces the s value with ‘__redacted__’)
- The tracking pixels then subsequently fire (FB and Google) and get the revised/redacted URL via JS
The above strategy can be applied to anything in the URL, but in this case, Query String parameters have been selected to redact.
Implementation
1. Set up a Custom JavaScript variable
The variable in GTM can be called anything – but in this example it will be called function - updateQsParameter
.
function() {
return function (uri, key, value) {
// remove the hash part before operating on the uri
var i = uri.indexOf('#');
var hash = i === -1 ? '' : uri.substr(i);
uri = i === -1 ? uri : uri.substr(0, i);
var re = new RegExp("([?&])" + key + "=.*?(&|$)", "i");
var separator = uri.indexOf('?') !== -1 ? "&" : "?";
if (value === null) {
// remove key-value pair if value is specifically null
uri = uri.replace(new RegExp("([?&]?)" + key + "=[^&]*", "i"), '');
if (uri.slice(-1) === '?') {
uri = uri.slice(0, -1);
}
// replace first occurrence of & by ? if no ? is present
if (uri.indexOf('?') === -1) uri = uri.replace(/&/, '?');
} else if (uri.match(re)) {
uri = uri.replace(re, '$1' + key + "=" + value + '$2');
}
return uri + hash;
}
}
Code modified from: https://gist.github.com/niyazpk/f8ac616f181f6042d1e0

2. Create a Tag to Call the redacting function
Create a Custom HTML tag with the following to call the redacting function “function – updateQsParameter”. The params_to_clean is an array of query string vars (line 2) you want to clean out and replace with the string “__redacted__” (line 5).
On Line 8, we use the history.replaceState
function to change the URL without redirecting the page. If you set the window.search parameter, as an example, it will make the browser redirect to the URL you are setting it to.
Fire the tag on the trigger “Initialization – All Pages” so that it fires before all marketing tags.
<script>
var params_to_clean = ["s"]
var new_url = {{Page URL}};
for (var i in params_to_clean) {
new_url = {{function - updateQsParameter}}(new_url, params_to_clean[i], "__redacted__");
}
window.history.replaceState({} , "", new_url);
</script>

Important: The order in which the code and tags run is extremely important. The redactor must run before any marketing tags fire. In the above example, it assumes that no marketing tags fire before the “Initialization – All Page” triggers
Check in Dev Tools that the redaction is being completed
So after publishing the changes or previewing them in GTM, we visit a URL like: https://mysite.com/search/?s=sensitiveinformationquery and we should see the pixels grabbing thue URL https://mysite.com/search/?s=__redacted__
We can check in Dev Tools to see what the pixels are picking up. In this example the Facebook Pixel and Google Analytics tag were being fired after the redaction. So I would expect to see the dl
parameter being updated with the redacted URL.
If we see this in Dev Tools’ Network
Panel we know the work has been done.
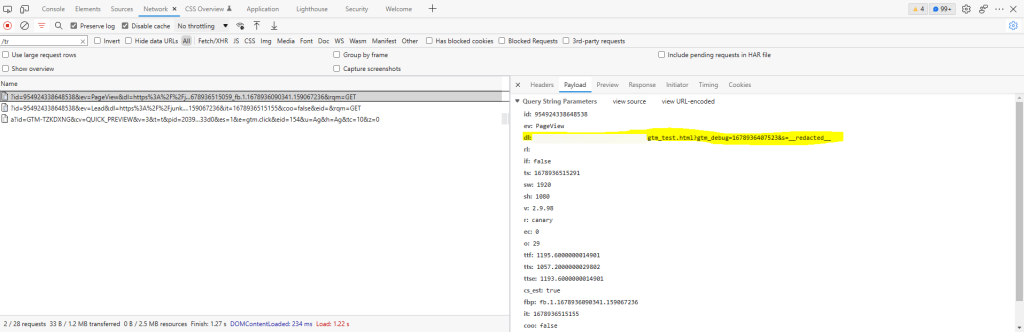

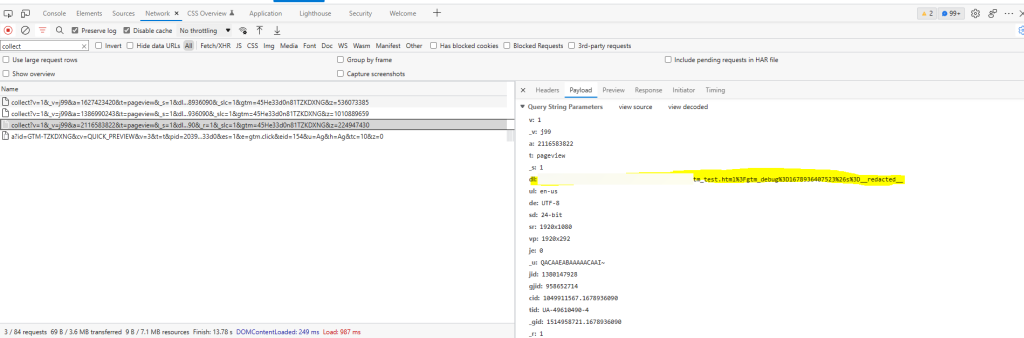